Even–odd rule
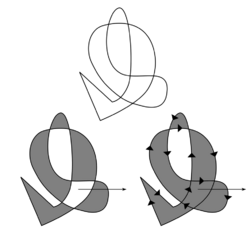
The even–odd rule is an algorithm implemented in vector-based graphic software,[1] like the PostScript language and Scalable Vector Graphics (SVG), which determines how a graphical shape with more than one closed outline will be filled. Unlike the nonzero-rule algorithm, this algorithm will alternatively color and leave uncolored shapes defined by nested closed paths irrespective of their winding.
The SVG defines the even–odd rule by saying:
This rule determines the "insideness" of a point on the canvas by drawing a ray from that point to infinity in any direction and counting the number of path segments from the given shape that the ray crosses. If this number is odd, the point is inside; if even, the point is outside.
The rule can be seen in effect in many vector graphic programs (such as Freehand or Illustrator), where a crossing of an outline with itself causes shapes to fill in strange ways.
On a simple curve, the even–odd rule reduces to a decision algorithm for the point in polygon problem.
The SVG computer graphics vector standard may be configured to use the even–odd rule when drawing polygons, though it uses the non-zero rule by default.[2]
Implementation
Below is a partial example implementation in Python:[3]
def is_point_in_path(x: int, y: int, poly: list[tuple[int, int]]) -> bool: """Determine if the point is on the path, corner, or boundary of the polygon Args: x -- The x coordinates of point. y -- The y coordinates of point. poly -- a list of tuples [(x, y), (x, y), ...] Returns: True if the point is in the path or is a corner or on the boundary""" num = len(poly) j = num - 1 c = False for i in range(num): if (x == poly[i][0]) and (y == poly[i][1]): # point is a corner return True if (poly[i][1] > y) != (poly[j][1] > y): slope = (x - poly[i][0]) * (poly[j][1] - poly[i][1]) - ( poly[j][0] - poly[i][0] ) * (y - poly[i][1]) if slope == 0: # point is on boundary return True if (slope < 0) != (poly[j][1] < poly[i][1]): c = not c j = i return c
See also
References
- ↑ J. D. Foley, A. van Dam, S. K. Feiner, and J. F. Hughes. Computer Graphics: Principles and Practice. The Systems Programming Series. Addison-Wesley, Reading, 2nd edition, 1990.
- ↑ [1], w3c.org, retrieved 2019-03-28
- ↑ "PNPOLY - Point Inclusion in Polygon Test - WR Franklin (WRF)". https://wrf.ecse.rpi.edu/Research/Short_Notes/pnpoly.html.
External links
![]() | Original source: https://en.wikipedia.org/wiki/Even–odd rule.
Read more |