LCP array
LCP array | ||||||||||
---|---|---|---|---|---|---|---|---|---|---|
Type | Array | |||||||||
Invented by | (Manber Myers) | |||||||||
Time complexity and space complexity in big O notation | ||||||||||
|
In computer science, the longest common prefix array (LCP array) is an auxiliary data structure to the suffix array. It stores the lengths of the longest common prefixes (LCPs) between all pairs of consecutive suffixes in a sorted suffix array.
For example, if A := [aab, ab, abaab, b, baab] is a suffix array, the longest common prefix between A[1] = aab and A[2] = ab is a which has length 1, so H[2] = 1 in the LCP array H. Likewise, the LCP of A[2] = ab and A[3] = abaab is ab, so H[3] = 2.
Augmenting the suffix array with the LCP array allows one to efficiently simulate top-down and bottom-up traversals of the suffix tree,[1][2] speeds up pattern matching on the suffix array[3] and is a prerequisite for compressed suffix trees.[4]
History
The LCP array was introduced in 1993, by Udi Manber and Gene Myers alongside the suffix array in order to improve the running time of their string search algorithm.[3]
Definition
Let [math]\displaystyle{ A }[/math] be the suffix array of the string [math]\displaystyle{ S=s_1,s_2,\ldots s_{n-1}\$ }[/math] of length [math]\displaystyle{ n }[/math], where [math]\displaystyle{ \$ }[/math] is a sentinel letter that is unique and lexicographically smaller than any other character. Let [math]\displaystyle{ S[i,j] }[/math] denote the substring of [math]\displaystyle{ S }[/math] ranging from [math]\displaystyle{ i }[/math] to [math]\displaystyle{ j }[/math]. Thus, [math]\displaystyle{ S[A[i], n] }[/math] is the [math]\displaystyle{ i }[/math]th smallest suffix of [math]\displaystyle{ S }[/math].
Let [math]\displaystyle{ \operatorname{lcp}(v,w) }[/math] denote the length of the longest common prefix between two strings [math]\displaystyle{ v }[/math] and [math]\displaystyle{ w }[/math]. Then the LCP array [math]\displaystyle{ H[1,n] }[/math] is an integer array of size [math]\displaystyle{ n }[/math] such that [math]\displaystyle{ H[1] }[/math] is undefined and [math]\displaystyle{ H[i]=\operatorname{lcp}(S[A[i-1],n],S[A[i],n]) }[/math] for every [math]\displaystyle{ 1\lt i\leq n }[/math]. Thus [math]\displaystyle{ H[i] }[/math] stores the length of longest common prefix of the lexicographically [math]\displaystyle{ i }[/math]th smallest suffix and its predecessor in the suffix array.
Difference between LCP array and suffix array:
- Suffix array: Represents the lexicographic rank of each suffix of an array.
- LCP array: Contains the maximum length prefix match between two consecutive suffixes, after they are sorted lexicographically.
Example
Consider the string [math]\displaystyle{ S = \textrm{banana\$} }[/math]:
i | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
---|---|---|---|---|---|---|---|
S[i] | b | a | n | a | n | a | $ |
and its corresponding sorted suffix array [math]\displaystyle{ A }[/math] :
i | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
---|---|---|---|---|---|---|---|
A[i] | 7 | 6 | 4 | 2 | 1 | 5 | 3 |
Suffix array with suffixes written out underneath vertically:
i | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
---|---|---|---|---|---|---|---|
A[i] | 7 | 6 | 4 | 2 | 1 | 5 | 3 |
S[A[i], n][1] | $ | a | a | a | b | n | n |
S[A[i], n][2] | $ | n | n | a | a | a | |
S[A[i], n][3] | a | a | n | $ | n | ||
S[A[i], n][4] | $ | n | a | a | |||
S[A[i], n][5] | a | n | $ | ||||
S[A[i], n][6] | $ | a | |||||
S[A[i], n][7] | $ |
Then the LCP array [math]\displaystyle{ H }[/math] is constructed by comparing lexicographically consecutive suffixes to determine their longest common prefix:
i | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
---|---|---|---|---|---|---|---|
H[i] | undefined | 0 | 1 | 3 | 0 | 0 | 2 |
So, for example, [math]\displaystyle{ H[4]=3 }[/math] is the length of the longest common prefix [math]\displaystyle{ \text{ana} }[/math] shared by the suffixes [math]\displaystyle{ A[3] = S[4,7] = \textrm{ana\$} }[/math] and [math]\displaystyle{ A[4] = S[2,7] = \textrm{anana\$} }[/math]. Note that [math]\displaystyle{ H[1] }[/math] is undefined, since there is no lexicographically smaller suffix.
Efficient construction algorithms
LCP array construction algorithms can be divided into two different categories: algorithms that compute the LCP array as a byproduct to the suffix array and algorithms that use an already constructed suffix array in order to compute the LCP values.
(Manber Myers) provide an algorithm to compute the LCP array alongside the suffix array in [math]\displaystyle{ O(n \log n) }[/math] time. (Kärkkäinen Sanders) show that it is also possible to modify their [math]\displaystyle{ O(n) }[/math] time algorithm such that it computes the LCP array as well. (Kasai Lee) present the first [math]\displaystyle{ O(n) }[/math] time algorithm (FLAAP) that computes the LCP array given the text and the suffix array.
Assuming that each text symbol takes one byte and each entry of the suffix or LCP array takes 4 bytes, the major drawback of their algorithm is a large space occupancy of [math]\displaystyle{ 13n }[/math] bytes, while the original output (text, suffix array, LCP array) only occupies [math]\displaystyle{ 9n }[/math] bytes. Therefore, (Manzini 2004) created a refined version of the algorithm of (Kasai Lee) (lcp9) and reduced the space occupancy to [math]\displaystyle{ 9n }[/math] bytes. (Kärkkäinen Manzini) provide another refinement of Kasai's algorithm ([math]\displaystyle{ \Phi }[/math]-algorithm) that improves the running time. Rather than the actual LCP array, this algorithm builds the permuted LCP (PLCP) array, in which the values appear in text order rather than lexicographical order.
(Gog Ohlebusch) provide two algorithms that although being theoretically slow ([math]\displaystyle{ O(n^2) }[/math]) were faster than the above-mentioned algorithms in practice.
(As of 2012), the currently fastest linear-time LCP array construction algorithm is due to (Fischer 2011), which in turn is based on one of the fastest suffix array construction algorithms (SA-IS) by (Nong Zhang). (Fischer Kurpicz) based on Yuta Mori's DivSufSort is even faster.
Applications
As noted by (Abouelhoda Kurtz) several string processing problems can be solved by the following kinds of tree traversals:
- bottom-up traversal of the complete suffix tree
- top-down traversal of a subtree of the suffix tree
- suffix tree traversal using the suffix links.
(Kasai Lee) show how to simulate a bottom-up traversal of the suffix tree using only the suffix array and LCP array. (Abouelhoda Kurtz) enhance the suffix array with the LCP array and additional data structures and describe how this enhanced suffix array can be used to simulate all three kinds of suffix tree traversals. (Fischer Heun) reduce the space requirements of the enhanced suffix array by preprocessing the LCP array for range minimum queries. Thus, every problem that can be solved by suffix tree algorithms can also be solved using the enhanced suffix array.[2]
Deciding if a pattern [math]\displaystyle{ P }[/math] of length [math]\displaystyle{ m }[/math] is a substring of a string [math]\displaystyle{ S }[/math] of length [math]\displaystyle{ n }[/math] takes [math]\displaystyle{ O(m \log n) }[/math] time if only the suffix array is used. By additionally using the LCP information, this bound can be improved to [math]\displaystyle{ O(m + \log n) }[/math] time.[3] (Abouelhoda Kurtz) show how to improve this running time even further to achieve optimal [math]\displaystyle{ O(m) }[/math] time. Thus, using suffix array and LCP array information, the decision query can be answered as fast as using the suffix tree.
The LCP array is also an essential part of compressed suffix trees which provide full suffix tree functionality like suffix links and lowest common ancestor queries.[5][6] Furthermore, it can be used together with the suffix array to compute the Lempel-Ziv LZ77 factorization in [math]\displaystyle{ O(n) }[/math] time.[2][7][8][9]
The longest repeated substring problem for a string [math]\displaystyle{ S }[/math] of length [math]\displaystyle{ n }[/math] can be solved in [math]\displaystyle{ \Theta(n) }[/math] time using both the suffix array [math]\displaystyle{ A }[/math] and the LCP array. It is sufficient to perform a linear scan through the LCP array in order to find its maximum value [math]\displaystyle{ v_{max} }[/math] and the corresponding index [math]\displaystyle{ i }[/math] where [math]\displaystyle{ v_{max} }[/math] is stored. The longest substring that occurs at least twice is then given by [math]\displaystyle{ S[A[i],A[i]+v_{max}-1] }[/math].
The remainder of this section explains two applications of the LCP array in more detail: How the suffix array and the LCP array of a string can be used to construct the corresponding suffix tree and how it is possible to answer LCP queries for arbitrary suffixes using range minimum queries on the LCP array.
Find the number of occurrences of a pattern
In order to find the number of occurrences of a given string [math]\displaystyle{ P }[/math] (length [math]\displaystyle{ m }[/math]) in a text [math]\displaystyle{ T }[/math] (length [math]\displaystyle{ N }[/math]),[3]
- We use binary search against the suffix array of [math]\displaystyle{ T }[/math] to find the starting and end position of all occurrences of [math]\displaystyle{ P }[/math].
- Now to speed up the search, we use LCP array, specifically a special version of the LCP array (LCP-LR below).
The issue with using standard binary search (without the LCP information) is that in each of the [math]\displaystyle{ O(\log N) }[/math] comparisons needed to be made, we compare P to the current entry of the suffix array, which means a full string comparison of up to m characters. So the complexity is [math]\displaystyle{ O(m\log N) }[/math].
The LCP-LR array helps improve this to [math]\displaystyle{ O(m+\log N) }[/math], in the following way:
At any point during the binary search algorithm, we consider, as usual, a range [math]\displaystyle{ (L,\dots,R) }[/math] of the suffix array and its central point [math]\displaystyle{ M }[/math], and decide whether we continue our search in the left sub-range [math]\displaystyle{ (L,\dots,M) }[/math] or in the right sub-range [math]\displaystyle{ (M,\dots,R) }[/math]. In order to make the decision, we compare [math]\displaystyle{ P }[/math] to the string at [math]\displaystyle{ M }[/math]. If [math]\displaystyle{ P }[/math] is identical to [math]\displaystyle{ M }[/math], our search is complete. But if not, we have already compared the first [math]\displaystyle{ k }[/math] characters of [math]\displaystyle{ P }[/math] and then decided whether [math]\displaystyle{ P }[/math] is lexicographically smaller or larger than [math]\displaystyle{ M }[/math]. Let's assume the outcome is that [math]\displaystyle{ P }[/math] is larger than [math]\displaystyle{ M }[/math]. So, in the next step, we consider [math]\displaystyle{ (M,\dots,R) }[/math] and a new central point [math]\displaystyle{ M' }[/math] in the middle:
M ...... M' ...... R | we know: lcp(P,M)==k
The trick now is that LCP-LR is precomputed such that an [math]\displaystyle{ O(1) }[/math]-lookup tells us the longest common prefix of [math]\displaystyle{ M }[/math] and [math]\displaystyle{ M' }[/math], [math]\displaystyle{ \mathrm{lcp}(M,M') }[/math].
We already know (from the previous step) that [math]\displaystyle{ M }[/math] itself has a prefix of [math]\displaystyle{ k }[/math] characters in common with [math]\displaystyle{ P }[/math]: [math]\displaystyle{ \mathrm{lcp}(P,M)=k }[/math]. Now there are three possibilities:
- Case 1: [math]\displaystyle{ k \lt \mathrm{lcp}(M,M') }[/math], i.e. [math]\displaystyle{ P }[/math] has fewer prefix characters in common with M than M has in common with M'. This means the (k+1)-th character of M' is the same as that of M, and since P is lexicographically larger than M, it must be lexicographically larger than M', too. So we continue in the right half (M',...,R).
- Case 2: [math]\displaystyle{ k \gt \mathrm{lcp}(M,M') }[/math], i.e. [math]\displaystyle{ P }[/math] has more prefix characters in common with [math]\displaystyle{ M }[/math] than [math]\displaystyle{ M }[/math] has in common with [math]\displaystyle{ M' }[/math]. Consequently, if we were to compare [math]\displaystyle{ P }[/math] to [math]\displaystyle{ M' }[/math], the common prefix would be smaller than [math]\displaystyle{ k }[/math], and [math]\displaystyle{ M' }[/math] would be lexicographically larger than [math]\displaystyle{ P }[/math], so, without actually making the comparison, we continue in the left half [math]\displaystyle{ (M,\dots,M') }[/math].
- Case 3: [math]\displaystyle{ k = \mathrm{lcp}(M,M') }[/math]. So M and M' are both identical with [math]\displaystyle{ P }[/math] in the first [math]\displaystyle{ k }[/math] characters. To decide whether we continue in the left or right half, it suffices to compare [math]\displaystyle{ P }[/math] to [math]\displaystyle{ M' }[/math] starting from the [math]\displaystyle{ (k+1) }[/math]th character.
- We continue recursively.
The overall effect is that no character of [math]\displaystyle{ P }[/math] is compared to any character of the text more than once. The total number of character comparisons is bounded by [math]\displaystyle{ m }[/math], so the total complexity is indeed [math]\displaystyle{ O(m+\log N) }[/math].
We still need to precompute LCP-LR so it is able to tell us in [math]\displaystyle{ O(1) }[/math] time the lcp between any two entries of the suffix array. We know the standard LCP array gives us the lcp of consecutive entries only, i.e. [math]\displaystyle{ \mathrm{lcp}(i-1,i) }[/math] for any [math]\displaystyle{ i }[/math]. However, [math]\displaystyle{ M }[/math] and [math]\displaystyle{ M' }[/math] in the description above are not necessarily consecutive entries.
The key to this is to realize that only certain ranges [math]\displaystyle{ (L,\dots,R) }[/math] will ever occur during the binary search: It always starts with [math]\displaystyle{ (0, \dots,N) }[/math] and divides that at the center, and then continues either left or right and divide that half again and so forth. Another way of looking at it is : every entry of the suffix array occurs as central point of exactly one possible range during binary search. So there are exactly N distinct ranges [math]\displaystyle{ (L\dots M\dots R) }[/math] that can possibly play a role during binary search, and it suffices to precompute [math]\displaystyle{ \mathrm{lcp}(L,M) }[/math] and [math]\displaystyle{ \mathrm{lcp}(M,R) }[/math] for those [math]\displaystyle{ N }[/math] possible ranges. So that is [math]\displaystyle{ 2N }[/math] distinct precomputed values, hence LCP-LR is [math]\displaystyle{ O(N) }[/math] in size.
Moreover, there is a straightforward recursive algorithm to compute the [math]\displaystyle{ 2N }[/math] values of LCP-LR in [math]\displaystyle{ O(N) }[/math] time from the standard LCP array.
To sum up:
- It is possible to compute LCP-LR in [math]\displaystyle{ O(N) }[/math] time and [math]\displaystyle{ O(2N)=O(N) }[/math] space from LCP.
- Using LCP-LR during binary search helps accelerate the search procedure from [math]\displaystyle{ O(M\log N) }[/math] to [math]\displaystyle{ O(M+\log N) }[/math].
- We can use two binary searches to determine the left and right end of the match range for [math]\displaystyle{ P }[/math], and the length of the match range corresponds with the number of occurrences for P.
Suffix tree construction
Given the suffix array [math]\displaystyle{ A }[/math] and the LCP array [math]\displaystyle{ H }[/math] of a string [math]\displaystyle{ S=s_1,s_2,\ldots s_n\$ }[/math] of length [math]\displaystyle{ n+1 }[/math], its suffix tree [math]\displaystyle{ ST }[/math] can be constructed in [math]\displaystyle{ O(n) }[/math] time based on the following idea: Start with the partial suffix tree for the lexicographically smallest suffix and repeatedly insert the other suffixes in the order given by the suffix array.
Let [math]\displaystyle{ ST_{i} }[/math] be the partial suffix tree for [math]\displaystyle{ 0\leq i \leq n }[/math]. Further let [math]\displaystyle{ d(v) }[/math] be the length of the concatenation of all path labels from the root of [math]\displaystyle{ ST_i }[/math] to node [math]\displaystyle{ v }[/math].
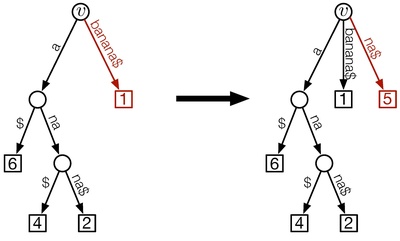
Start with [math]\displaystyle{ ST_0 }[/math], the tree consisting only of the root. To insert [math]\displaystyle{ A[i+1] }[/math] into [math]\displaystyle{ ST_i }[/math], walk up the rightmost path beginning at the recently inserted leaf [math]\displaystyle{ A[i] }[/math] to the root, until the deepest node [math]\displaystyle{ v }[/math] with [math]\displaystyle{ d(v) \leq H[i+1] }[/math] is reached.
We need to distinguish two cases:
- [math]\displaystyle{ d(v)=H[i+1] }[/math]: This means that the concatenation of the labels on the root-to-[math]\displaystyle{ v }[/math] path equals the longest common prefix of suffixes [math]\displaystyle{ A[i] }[/math] and [math]\displaystyle{ A[i+1] }[/math].
In this case, insert [math]\displaystyle{ A[i+1] }[/math] as a new leaf [math]\displaystyle{ x }[/math] of node [math]\displaystyle{ v }[/math] and label the edge [math]\displaystyle{ (v,x) }[/math] with [math]\displaystyle{ S[A[i+1]+H[i+1],n] }[/math]. Thus the edge label consists of the remaining characters of suffix [math]\displaystyle{ A[i+1] }[/math] that are not already represented by the concatenation of the labels of the root-to-[math]\displaystyle{ v }[/math] path.
This creates the partial suffix tree [math]\displaystyle{ ST_{i+1} }[/math].Case 2 ([math]\displaystyle{ d(v) \lt H[i+1] }[/math]): In order to add suffix [math]\displaystyle{ nana\$ }[/math], the edge to the previously inserted suffix [math]\displaystyle{ na\$ }[/math] has to be split up. The new edge to the new internal node is labeled with the longest common prefix of the suffixes [math]\displaystyle{ na$ }[/math] and [math]\displaystyle{ nana\$ }[/math]. The edges connecting the two leaves are labeled with the remaining suffix characters that are not part of the prefix. - [math]\displaystyle{ d(v) \lt H[i+1] }[/math]: This means that the concatenation of the labels on the root-to-[math]\displaystyle{ v }[/math] path displays less characters than the longest common prefix of suffixes [math]\displaystyle{ A[i] }[/math] and [math]\displaystyle{ A[i+1] }[/math] and the missing characters are contained in the edge label of [math]\displaystyle{ v }[/math]'s rightmost edge. Therefore, we have to split up that edge as follows:
Let [math]\displaystyle{ w }[/math] be the child of [math]\displaystyle{ v }[/math] on [math]\displaystyle{ ST_i }[/math]'s rightmost path.
- Delete the edge [math]\displaystyle{ (v,w) }[/math].
- Add a new internal node [math]\displaystyle{ y }[/math] and a new edge [math]\displaystyle{ (v,y) }[/math] with label [math]\displaystyle{ S[A[i]+d(v),A[i]+H[i+1]-1] }[/math]. The new label consists of the missing characters of the longest common prefix of [math]\displaystyle{ A[i] }[/math] and [math]\displaystyle{ A[i+1] }[/math]. Thus, the concatenation of the labels of the root-to-[math]\displaystyle{ y }[/math] path now displays the longest common prefix of [math]\displaystyle{ A[i] }[/math] and [math]\displaystyle{ A[i+1] }[/math].
- Connect [math]\displaystyle{ w }[/math] to the newly created internal node [math]\displaystyle{ y }[/math] by an edge [math]\displaystyle{ (y,w) }[/math] that is labeled [math]\displaystyle{ S[A[i]+H[i+1],A[i]+d(w)-1] }[/math]. The new label consists of the remaining characters of the deleted edge [math]\displaystyle{ (v,w) }[/math] that were not used as the label of edge [math]\displaystyle{ (v,y) }[/math].
- Add [math]\displaystyle{ A[i+1] }[/math] as a new leaf [math]\displaystyle{ x }[/math] and connect it to the new internal node [math]\displaystyle{ y }[/math] by an edge [math]\displaystyle{ (y,x) }[/math] that is labeled [math]\displaystyle{ S[A[i+1]+H[i+1],n] }[/math]. Thus the edge label consists of the remaining characters of suffix [math]\displaystyle{ A[i+1] }[/math] that are not already represented by the concatenation of the labels of the root-to-[math]\displaystyle{ v }[/math] path.
- This creates the partial suffix tree [math]\displaystyle{ ST_{i+1} }[/math].
A simple amortization argument shows that the running time of this algorithm is bounded by [math]\displaystyle{ O(n) }[/math]:
The nodes that are traversed in step [math]\displaystyle{ i }[/math] by walking up the rightmost path of [math]\displaystyle{ ST_i }[/math] (apart from the last node [math]\displaystyle{ v }[/math]) are removed from the rightmost path, when [math]\displaystyle{ A[i+1] }[/math] is added to the tree as a new leaf. These nodes will never be traversed again for all subsequent steps [math]\displaystyle{ j\gt i }[/math]. Therefore, at most [math]\displaystyle{ 2n }[/math] nodes will be traversed in total.
LCP queries for arbitrary suffixes
The LCP array [math]\displaystyle{ H }[/math] only contains the length of the longest common prefix of every pair of consecutive suffixes in the suffix array [math]\displaystyle{ A }[/math]. However, with the help of the inverse suffix array [math]\displaystyle{ A^{-1} }[/math] ([math]\displaystyle{ A[i]= j \Leftrightarrow A^{-1}[j]= i }[/math], i.e. the suffix [math]\displaystyle{ S[j,n] }[/math] that starts at position [math]\displaystyle{ j }[/math] in [math]\displaystyle{ S }[/math] is stored in position [math]\displaystyle{ A^{-1}[j] }[/math] in [math]\displaystyle{ A }[/math]) and constant-time range minimum queries on [math]\displaystyle{ H }[/math], it is possible to determine the length of the longest common prefix of arbitrary suffixes in [math]\displaystyle{ O(1) }[/math] time.
Because of the lexicographic order of the suffix array, every common prefix of the suffixes [math]\displaystyle{ S[i,n] }[/math] and [math]\displaystyle{ S[j,n] }[/math] has to be a common prefix of all suffixes between [math]\displaystyle{ i }[/math]'s position in the suffix array [math]\displaystyle{ A^{-1}[i] }[/math] and [math]\displaystyle{ j }[/math]'s position in the suffix array [math]\displaystyle{ A^{-1}[j] }[/math]. Therefore, the length of the longest prefix that is shared by all of these suffixes is the minimum value in the interval [math]\displaystyle{ H[A^{-1}[i]+1,A^{-1}[j]] }[/math]. This value can be found in constant time if [math]\displaystyle{ H }[/math] is preprocessed for range minimum queries.
Thus given a string [math]\displaystyle{ S }[/math] of length [math]\displaystyle{ n }[/math] and two arbitrary positions [math]\displaystyle{ i,j }[/math] in the string [math]\displaystyle{ S }[/math] with [math]\displaystyle{ A^{-1}[i] \lt A^{-1}[j] }[/math], the length of the longest common prefix of the suffixes [math]\displaystyle{ S[i,n] }[/math] and [math]\displaystyle{ S[j,n] }[/math] can be computed as follows: [math]\displaystyle{ \operatorname{LCP}(i,j)=H[\operatorname{RMQ}_H(A^{-1}[i]+1,A^{-1}[j])] }[/math].
Notes
References
- Abouelhoda, Mohamed Ibrahim; Kurtz, Stefan; Ohlebusch, Enno (2004). "Replacing suffix trees with enhanced suffix arrays". Journal of Discrete Algorithms 2: 53–86. doi:10.1016/S1570-8667(03)00065-0.
- Manber, Udi; Myers, Gene (1993). "Suffix Arrays: A New Method for On-Line String Searches". SIAM Journal on Computing 22 (5): 935. doi:10.1137/0222058.
- Kasai, T.; Lee, G.; Arimura, H.; Arikawa, S.; Park, K. (2001). "Linear-Time Longest-Common-Prefix Computation in Suffix Arrays and Its Applications". Proceedings of the 12th Annual Symposium on Combinatorial Pattern Matching. 2089. pp. 181–192. doi:10.1007/3-540-48194-X_17. ISBN 978-3-540-42271-6.
- Ohlebusch, Enno; Fischer, Johannes; Gog, Simon (2010). "CST++". String Processing and Information Retrieval. 6393. pp. 322. doi:10.1007/978-3-642-16321-0_34. ISBN 978-3-642-16320-3.
- Kärkkäinen, Juha (2003). "Simple linear work suffix array construction". Proceedings of the 30th international conference on Automata, languages and programming. pp. 943–955. http://dl.acm.org/citation.cfm?id=1759210.1759301. Retrieved 2012-08-28.
- Fischer, Johannes (2011). "Inducing the LCP-Array". Algorithms and Data Structures. 6844. pp. 374–385. doi:10.1007/978-3-642-22300-6_32. ISBN 978-3-642-22299-3.
- Manzini, Giovanni (2004). "Two Space Saving Tricks for Linear Time LCP Array Computation". Algorithm Theory – SWAT 2004. 3111. pp. 372. doi:10.1007/978-3-540-27810-8_32. ISBN 978-3-540-22339-9.
- Kärkkäinen, Juha; Manzini, Giovanni; Puglisi, Simon J. (2009). "Permuted Longest-Common-Prefix Array". Combinatorial Pattern Matching. 5577. pp. 181. doi:10.1007/978-3-642-02441-2_17. ISBN 978-3-642-02440-5.
- Puglisi, Simon J.; Turpin, Andrew (2008). "Space-Time Tradeoffs for Longest-Common-Prefix Array Computation". Algorithms and Computation. 5369. pp. 124. doi:10.1007/978-3-540-92182-0_14. ISBN 978-3-540-92181-3.
- Gog, Simon; Ohlebusch, Enno (2011). "Fast and Lightweight LCP-Array Construction Algorithms". Proceedings of the Workshop on Algorithm Engineering and Experiments, ALENEX 2011. pp. 25–34. http://www.siam.org/proceedings/alenex/2011/alx11_03_gogs.pdf. Retrieved 2012-08-28.
- Nong, Ge; Zhang, Sen; Chan, Wai Hong (2009). "Linear Suffix Array Construction by Almost Pure Induced-Sorting". 2009 Data Compression Conference. pp. 193. doi:10.1109/DCC.2009.42. ISBN 978-0-7695-3592-0.
- Fischer, Johannes; Heun, Volker (2007). "A New Succinct Representation of RMQ-Information and Improvements in the Enhanced Suffix Array". Combinatorics, Algorithms, Probabilistic and Experimental Methodologies. 4614. pp. 459. doi:10.1007/978-3-540-74450-4_41. ISBN 978-3-540-74449-8.
- Chen, G.; Puglisi, S. J.; Smyth, W. F. (2008). "Lempel–Ziv Factorization Using Less Time & Space". Mathematics in Computer Science 1 (4): 605. doi:10.1007/s11786-007-0024-4.
- Crochemore, M.; Ilie, L. (2008). "Computing Longest Previous Factor in linear time and applications". Information Processing Letters 106 (2): 75. doi:10.1016/j.ipl.2007.10.006.
- Crochemore, M.; Ilie, L.; Smyth, W. F. (2008). "A Simple Algorithm for Computing the Lempel Ziv Factorization". Data Compression Conference (dcc 2008). pp. 482. doi:10.1109/DCC.2008.36. ISBN 978-0-7695-3121-2.
- Sadakane, K. (2007). "Compressed Suffix Trees with Full Functionality". Theory of Computing Systems 41 (4): 589–607. doi:10.1007/s00224-006-1198-x.
- Fischer, Johannes; Mäkinen, Veli; Navarro, Gonzalo (2009). "Faster entropy-bounded compressed suffix trees". Theoretical Computer Science 410 (51): 5354. doi:10.1016/j.tcs.2009.09.012.
- Fischer, Johannes; Kurpicz, Florian (5 October 2017). "Dismantling DivSufSort". Proceedings of the Prague Stringology Conference 2017.
External links
- Mirror of the ad-hoc-implementation of the code described in (Fischer 2011)
- SDSL: Succinct Data Structure Library - Provides various LCP array implementations, Range Minimum Query (RMQ) support structures and many more succinct data structures
- Bottom-up suffix tree traversal emulated using suffix array and LCP array (Java)
- Text-Indexing project (linear-time construction of suffix trees, suffix arrays, LCP array and Burrows–Wheeler Transform)
![]() | Original source: https://en.wikipedia.org/wiki/LCP array.
Read more |