Maximum subarray problem
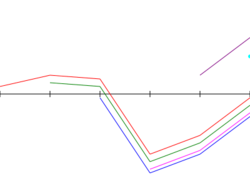
In computer science, the maximum sum subarray problem, also known as the maximum segment sum problem, is the task of finding a contiguous subarray with the largest sum, within a given one-dimensional array A[1...n] of numbers. It can be solved in [math]\displaystyle{ O(n) }[/math] time and [math]\displaystyle{ O(1) }[/math] space.
Formally, the task is to find indices [math]\displaystyle{ i }[/math] and [math]\displaystyle{ j }[/math] with [math]\displaystyle{ 1 \leq i \leq j \leq n }[/math], such that the sum
- [math]\displaystyle{ \sum_{x=i}^j A[x] }[/math]
is as large as possible. (Some formulations of the problem also allow the empty subarray to be considered; by convention, the sum of all values of the empty subarray is zero.) Each number in the input array A could be positive, negative, or zero.[1]
For example, for the array of values [−2, 1, −3, 4, −1, 2, 1, −5, 4], the contiguous subarray with the largest sum is [4, −1, 2, 1], with sum 6.
Some properties of this problem are:
- If the array contains all non-negative numbers, then the problem is trivial; a maximum subarray is the entire array.
- If the array contains all non-positive numbers, then a solution is any subarray of size 1 containing the maximal value of the array (or the empty subarray, if it is permitted).
- Several different sub-arrays may have the same maximum sum.
Although this problem can be solved using several different algorithmic techniques, including brute force,[2] divide and conquer,[3] dynamic programming,[4] and reduction to shortest paths, a simple single-pass algorithm known as Kadane's algorithm solves it efficiently.
History
The maximum subarray problem was proposed by Ulf Grenander in 1977 as a simplified model for maximum likelihood estimation of patterns in digitized images.[5]
Grenander was looking to find a rectangular subarray with maximum sum, in a two-dimensional array of real numbers. A brute-force algorithm for the two-dimensional problem runs in O(n6) time; because this was prohibitively slow, Grenander proposed the one-dimensional problem to gain insight into its structure. Grenander derived an algorithm that solves the one-dimensional problem in O(n2) time,[note 1] improving the brute force running time of O(n3). When Michael Shamos heard about the problem, he overnight devised an O(n log n) divide-and-conquer algorithm for it. Soon after, Shamos described the one-dimensional problem and its history at a Carnegie Mellon University seminar attended by Jay Kadane, who designed within a minute an O(n)-time algorithm,[5][6][7] which is as fast as possible.[note 2] In 1982, David Gries obtained the same O(n)-time algorithm by applying Dijkstra's "standard strategy";[8] in 1989, Richard Bird derived it by purely algebraic manipulation of the brute-force algorithm using the Bird–Meertens formalism.[9]
Grenander's two-dimensional generalization can be solved in O(n3) time either by using Kadane's algorithm as a subroutine, or through a divide-and-conquer approach. Slightly faster algorithms based on distance matrix multiplication have been proposed by (Tamaki Tokuyama) and by (Takaoka 2002). There is some evidence that no significantly faster algorithm exists; an algorithm that solves the two-dimensional maximum subarray problem in O(n3−ε) time, for any ε>0, would imply a similarly fast algorithm for the all-pairs shortest paths problem.[10]
Applications
Maximum subarray problems arise in many fields, such as genomic sequence analysis and computer vision.
Genomic sequence analysis employs maximum subarray algorithms to identify important biological segments of protein sequences.[citation needed] These problems include conserved segments, GC-rich regions, tandem repeats, low-complexity filter, DNA binding domains, and regions of high charge.[citation needed]
In computer vision, maximum-subarray algorithms are used on bitmap images to detect the brightest area in an image.
Kadane's algorithm
No empty subarrays admitted
Kadane's algorithm scans the given array [math]\displaystyle{ A[1\ldots n] }[/math] from left to right.
In the [math]\displaystyle{ j }[/math]th step, it computes the subarray with the largest sum ending at [math]\displaystyle{ j }[/math]; this sum is maintained in variable current_sum
.[note 3]
Moreover, it computes the subarray with the largest sum anywhere in [math]\displaystyle{ A[1 \ldots j] }[/math], maintained in variable best_sum
,[note 4]
and easily obtained as the maximum of all values of current_sum
seen so far, cf. line 7 of the algorithm.
As a loop invariant, in the [math]\displaystyle{ j }[/math]th step, the old value of current_sum
holds the maximum over all [math]\displaystyle{ i \in \{ 1,\ldots, j-1 \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j-1] }[/math].
Therefore, current_sum
[math]\displaystyle{ +A[j] }[/math][note 5]
is the maximum over all [math]\displaystyle{ i \in \{ 1,\ldots, j-1 \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j] }[/math]. To extend the latter maximum to cover also the case [math]\displaystyle{ i=j }[/math], it is sufficient to consider also the singleton subarray [math]\displaystyle{ A[j \; \ldots \; j] }[/math]. This is done in line 6 by assigning [math]\displaystyle{ \max(A[j], }[/math]current_sum
[math]\displaystyle{ +A[j]) }[/math] as the new value of current_sum
, which after that holds the maximum over all [math]\displaystyle{ i \in \{ 1, \ldots, j \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j] }[/math].
Thus, the problem can be solved with the following code,[11] expressed in Python.
def max_subarray(numbers): """Find the largest sum of any contiguous subarray.""" best_sum = - infinity current_sum = 0 for x in numbers: current_sum = max(x, current_sum + x) best_sum = max(best_sum, current_sum) return best_sum
If the input contains no positive element, the returned value is that of the largest element (i.e., the value closest to 0), or negative infinity if the input was empty. For correctness, an exception should be raised when the input array is empty, since an empty array has no maximum nonempty subarray. If the array is nonempty, its first element could be used in place of negative infinity, if needed to avoid mixing numeric and non-numeric values.
The algorithm can be adapted to the case which allows empty subarrays or to keep track of the starting and ending indices of the maximum subarray.
This algorithm calculates the maximum subarray ending at each position from the maximum subarray ending at the previous position, so it can be viewed as a trivial case of dynamic programming.
Empty subarrays admitted
Kadane's original algorithm solves the problem variant when empty subarrays are admitted.[4][7]
This variant will return 0 if the input contains no positive elements (including when the input is empty).
It is obtained by two changes in code: in line 3, best_sum
should be initialized to 0 to account for the empty subarray [math]\displaystyle{ A[0 \ldots -1] }[/math]
best_sum = 0;
and line 6 in the for loop current_sum
should be updated as max(0, current_sum + x)
.[note 6]
current_sum = max(0, current_sum + x)
As a loop invariant, in the [math]\displaystyle{ j }[/math]th step, the old value of current_sum
holds the maximum over all [math]\displaystyle{ i \in \{ 1,\ldots, j \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j-1] }[/math].[note 7]
Therefore, current_sum
[math]\displaystyle{ +A[j] }[/math]
is the maximum over all [math]\displaystyle{ i \in \{ 1,\ldots, j \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j] }[/math]. To extend the latter maximum to cover also the case [math]\displaystyle{ i=j+1 }[/math], it is sufficient to consider also the empty subarray [math]\displaystyle{ A[j+1 \; \ldots \; j] }[/math]. This is done in line 6 by assigning [math]\displaystyle{ \max(0, }[/math]current_sum
[math]\displaystyle{ +A[j]) }[/math] as the new value of current_sum
, which after that holds the maximum over all [math]\displaystyle{ i \in \{ 1, \ldots, j+1 \} }[/math] of the sum [math]\displaystyle{ A[i]+\cdots+A[j] }[/math]. Machine-verified C / Frama-C code of both variants can be found here.
Computing the best subarray's position
The algorithm can be modified to keep track of the starting and ending indices of the maximum subarray as well.
Because of the way this algorithm uses optimal substructures (the maximum subarray ending at each position is calculated in a simple way from a related but smaller and overlapping subproblem: the maximum subarray ending at the previous position) this algorithm can be viewed as a simple/trivial example of dynamic programming.
Complexity
The runtime complexity of Kadane's algorithm is [math]\displaystyle{ O(n) }[/math] and its space complexity is [math]\displaystyle{ O(1) }[/math].[4][7]
Generalizations
Similar problems may be posed for higher-dimensional arrays, but their solutions are more complicated; see, e.g., (Takaoka 2002). (Brodal Jørgensen) showed how to find the k largest subarray sums in a one-dimensional array, in the optimal time bound [math]\displaystyle{ O(n + k) }[/math].
The Maximum sum k-disjoint subarrays can also be computed in the optimal time bound [math]\displaystyle{ O(n + k) }[/math] .[12]
See also
Notes
- ↑ By using a precomputed table of cumulative sums [math]\displaystyle{ S[k] = \sum_{x=1}^k A[x] }[/math] to compute the subarray sum [math]\displaystyle{ \sum_{x=i}^j A[x] = S[j] - S[i-1] }[/math] in constant time
- ↑ since every algorithm must at least scan the array once which already takes O(n) time
- ↑ named
MaxEndingHere
in (Bentley 1989), andc
in (Gries 1982) - ↑ named
MaxSoFar
in (Bentley 1989), ands
in (Gries 1982) - ↑
In the Python code below, [math]\displaystyle{ A[j] }[/math] is expressed as
x
, with the index [math]\displaystyle{ j }[/math] left implicit. - ↑ While (Bentley 1989) does not mention this difference, using
x
instead of0
in the above version without empty subarrays achieves maintaining its loop invariantcurrent_sum
[math]\displaystyle{ =\max_{i \in \{ 1, ..., j-1 \}} A[i]+...+A[j-1] }[/math] at the beginning of the [math]\displaystyle{ j }[/math]th step. - ↑ This sum is [math]\displaystyle{ 0 }[/math] when [math]\displaystyle{ i=j }[/math], corresponding to the empty subarray [math]\displaystyle{ A[j\ldots j-1] }[/math].
References
- ↑ Bentley 1989, p. 69.
- ↑ Bentley 1989, p. 70.
- ↑ Bentley 1989, p. 73.
- ↑ Jump up to: 4.0 4.1 4.2 Bentley 1989, p. 74.
- ↑ Jump up to: 5.0 5.1 Bentley 1984, p. 868-869.
- ↑ Bentley 1989, p. 76-77.
- ↑ Jump up to: 7.0 7.1 7.2 Gries 1982, p. 211.
- ↑ Gries 1982, p. 209-211.
- ↑ Bird 1989, Sect.8, p.126.
- ↑ Backurs, Dikkala & Tzamos 2016.
- ↑ Bentley 1989, p. 78,171. Bentley, like Gries, first introduces the variant admitting empty subarrays, see below, and describes only the changes.
- ↑ Bengtsson & Chen 2007.
- Backurs, Arturs; Dikkala, Nishanth; Tzamos, Christos (2016), "Tight Hardness Results for Maximum Weight Rectangles", Proc. 43rd International Colloquium on Automata, Languages, and Programming: 81:1–81:13, doi:10.4230/LIPIcs.ICALP.2016.81
- Bae, Sung Eun (2007), Sequential and Parallel Algorithms for the Generalized Maximum Subarray Problem, https://pdfs.semanticscholar.org/bea4/1795adaf240b9db4195b9dc511bd8d46bff1.pdf.
- Bengtsson, Fredrik; Chen, Jingsen (2007), Computing maximum-scoring segments optimally, http://ltu.diva-portal.org/smash/get/diva2:995901/FULLTEXT01.pdf
- Bentley, Jon (1984), "Programming Pearls: Algorithm Design Techniques", Communications of the ACM 27 (9): 865–873, doi:10.1145/358234.381162
- Bentley, Jon (May 1989), Programming Pearls (2nd? ed.), Reading, MA: Addison Wesley, ISBN 0-201-10331-1, https://archive.org/details/programmingpearl00bent
- {{citation
| url=http://comjnl.oxfordjournals.org/content/32/2/122.full.pdf | first=Richard S. | last= Bird | title=Algebraic Identities for Program Calculation | journal=The Computer Journal | volume=32 | number=2 | pages=122–126 | year=1989 | doi=10.1093/comjnl/32.2.122
- Brodal, Gerth Stølting; Jørgensen, Allan Grønlund (2007), "A linear time algorithm for the k maximal sums problem", Mathematical Foundations of Computer Science 2007, Lecture Notes in Computer Science, 4708, Springer-Verlag, pp. 442–453, doi:10.1007/978-3-540-74456-6_40, ISBN 978-3-540-74455-9.
- Gries, David (1982), "A Note on the Standard Strategy for Developing Loop Invariants and Loops", Science of Computer Programming 2 (3): 207–241, doi:10.1016/0167-6423(83)90015-1, https://core.ac.uk/download/pdf/82596333.pdf
- Takaoka, Tadao (2002), "Efficient algorithms for the maximum subarray problem by distance matrix multiplication", Electronic Notes in Theoretical Computer Science 61: 191–200, doi:10.1016/S1571-0661(04)00313-5.
- Tamaki, Hisao; Tokuyama, Takeshi (1998), "Algorithms for the Maximum Subarray Problem Based on Matrix Multiplication", Proceedings of the 9th Symposium on Discrete Algorithms (SODA): 446–452, http://dl.acm.org/citation.cfm?id=314613.314823, retrieved November 17, 2018
External links
- TAN, Lirong. "Maximum Contiguous Subarray Sum Problems". http://www.picb.ac.cn/~xiaohang/vimwiki/study/tanlirong/Algorithm/project/Report.pdf.
- Mu, Shin-Cheng (2010). "The Maximum Segment Sum Problem: Its Origin, and a Derivation". https://www.iis.sinica.edu.tw/~scm/2010/maximum-segment-sum-origin-and-derivation.
- "Notes on Maximum Subarray Problem". 2012. http://cs.slu.edu/~goldwamh/courses/slu/csci314/2012_Fall/lectures/maxsubarray/.
- www.algorithmist.com
- alexeigor.wikidot.com
- greatest subsequential sum problem on Rosetta Code
- geeksforgeeks page on Kadane's Algorithm
![]() | Original source: https://en.wikipedia.org/wiki/Maximum subarray problem.
Read more |