Greedy coloring
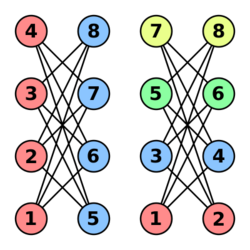
In the study of graph coloring problems in mathematics and computer science, a greedy coloring or sequential coloring[1] is a coloring of the vertices of a graph formed by a greedy algorithm that considers the vertices of the graph in sequence and assigns each vertex its first available color. Greedy colorings can be found in linear time, but they do not, in general, use the minimum number of colors possible.
Different choices of the sequence of vertices will typically produce different colorings of the given graph, so much of the study of greedy colorings has concerned how to find a good ordering. There always exists an ordering that produces an optimal coloring, but although such orderings can be found for many special classes of graphs, they are hard to find in general. Commonly used strategies for vertex ordering involve placing higher-degree vertices earlier than lower-degree vertices, or choosing vertices with fewer available colors in preference to vertices that are less constrained.
Variations of greedy coloring choose the colors in an online manner, without any knowledge of the structure of the uncolored part of the graph, or choose other colors than the first available in order to reduce the total number of colors. Greedy coloring algorithms have been applied to scheduling and register allocation problems, the analysis of combinatorial games, and the proofs of other mathematical results including Brooks' theorem on the relation between coloring and degree. Other concepts in graph theory derived from greedy colorings include the Grundy number of a graph (the largest number of colors that can be found by a greedy coloring), and the well-colored graphs, graphs for which all greedy colorings use the same number of colors.
Algorithm
The greedy coloring for a given vertex ordering can be computed by an algorithm that runs in linear time. The algorithm processes the vertices in the given ordering, assigning a color to each one as it is processed. The colors may be represented by the numbers [math]\displaystyle{ 0,1,2,\dots }[/math] and each vertex is given the color with the smallest number that is not already used by one of its neighbors. To find the smallest available color, one may use an array to count the number of neighbors of each color (or alternatively, to represent the set of colors of neighbors), and then scan the array to find the index of its first zero.[2]
In Python, the algorithm can be expressed as:
def first_available(color_list): """Return smallest non-negative integer not in the given list of colors.""" color_set = set(color_list) count = 0 while True: if count not in color_set: return count count += 1 def greedy_color(G, order): """Find the greedy coloring of G in the given order. The representation of G is assumed to be like https://www.python.org/doc/essays/graphs/ in allowing neighbors of a node/vertex to be iterated over by "for w in G[node]". The return value is a dictionary mapping vertices to their colors.""" color = dict() for node in order: used_neighbour_colors = [color[nbr] for nbr in G[node] if nbr in color] color[node] = first_available(used_neighbour_colors) return color
The first_available
subroutine takes time proportional to the length of its argument list, because it performs two loops, one over the list itself and one over a list of counts that has the same length. The time for the overall coloring algorithm is dominated by the calls to this subroutine. Each edge in the graph contributes to only one of these calls, the one for the endpoint of the edge that is later in the vertex ordering. Therefore, the sum of the lengths of the argument lists to first_available
, and the total time for the algorithm, are proportional to the number of edges in the graph.[2]
An alternative algorithm, producing the same coloring,[3] is to choose the sets of vertices with each color, one color at a time. In this method, each color class [math]\displaystyle{ C }[/math] is chosen by scanning through the vertices in the given ordering. When this scan encounters an uncolored vertex [math]\displaystyle{ v }[/math] that has no neighbor in [math]\displaystyle{ C }[/math], it adds [math]\displaystyle{ v }[/math] to [math]\displaystyle{ C }[/math]. In this way, [math]\displaystyle{ C }[/math] becomes a maximal independent set among the vertices that were not already assigned smaller colors. The algorithm repeatedly finds color classes in this way until all vertices are colored. However, it involves making multiple scans of the graph, one scan for each color class, instead of the method outlined above which uses only a single scan.[4]
Choice of ordering
Different orderings of the vertices of a graph may cause the greedy coloring to use different numbers of colors, ranging from the optimal number of colors to, in some cases, a number of colors that is proportional to the number of vertices in the graph. For instance, a crown graph (a graph formed from two disjoint sets of n/2 vertices {a1, a2, ...} and {b1, b2, ...} by connecting ai to bj whenever i ≠ j) can be a particularly bad case for greedy coloring. With the vertex ordering a1, b1, a2, b2, ..., a greedy coloring will use n/2 colors, one color for each pair (ai, bi). However, the optimal number of colors for this graph is two, one color for the vertices ai and another for the vertices bi.[5] There also exist graphs such that with high probability a randomly chosen vertex ordering leads to a number of colors much larger than the minimum.[6] Therefore, it is of some importance in greedy coloring to choose the vertex ordering carefully.
Good orders
The vertices of any graph may always be ordered in such a way that the greedy algorithm produces an optimal coloring. For, given any optimal coloring, one may order the vertices by their colors. Then when one uses a greedy algorithm with this order, the resulting coloring is automatically optimal.[7] However, because optimal graph coloring is NP-complete, any subproblem that would allow this problem to be solved quickly, including finding an optimal ordering for greedy coloring, is NP-hard.[8]
In interval graphs and chordal graphs, if the vertices are ordered in the reverse of a perfect elimination ordering, then the earlier neighbors of every vertex will form a clique. This property causes the greedy coloring to produce an optimal coloring, because it never uses more colors than are required for each of these cliques. An elimination ordering can be found in linear time, when it exists.[9]
More strongly, any perfect elimination ordering is hereditarily optimal, meaning that it is optimal both for the graph itself and for all of its induced subgraphs. The perfectly orderable graphs (which include chordal graphs, comparability graphs, and distance-hereditary graphs) are defined as graphs that have a hereditarily optimal ordering.[10] Recognizing perfectly orderable graphs is also NP-complete.[11]
Bad orders
The number of colors produced by the greedy coloring for the worst ordering of a given graph is called its Grundy number.[12] Just as finding a good vertex ordering for greedy coloring is difficult, so is finding a bad vertex ordering. It is NP-complete to determine, for a given graph G and number k, whether there exists an ordering of the vertices of G that causes the greedy algorithm to use k or more colors. In particular, this means that it is difficult to find the worst ordering for G.[12]
Graphs for which order is irrelevant
The well-colored graphs are the graphs for which all vertex orderings produce the same number of colors. This number of colors, in these graphs, equals both the chromatic number and the Grundy number.[12] They include the cographs, which are exactly the graphs in which all induced subgraphs are well-colored.[13] However, it is co-NP-complete to determine whether a graph is well-colored.[12]
If a random graph is drawn from the Erdős–Rényi model with constant probability of including each edge, then any vertex ordering that is chosen independently of the graph edges leads to a coloring whose number of colors is close to twice the optimal value, with high probability. It remains unknown whether there is any polynomial time method for finding significantly better colorings of these graphs.[3]
Degeneracy
Because optimal vertex orderings are hard to find, heuristics have been used that attempt to reduce the number of colors while not guaranteeing an optimal number of colors. A commonly used ordering for greedy coloring is to choose a vertex v of minimum degree, order the subgraph with v removed recursively, and then place v last in the ordering. The largest degree of a removed vertex that this algorithm encounters is called the degeneracy of the graph, denoted d. In the context of greedy coloring, the same ordering strategy is also called the smallest last ordering.[14] This vertex ordering, and the degeneracy, may be computed in linear time.[15] It can be viewed as an improved version of an earlier vertex ordering method, the largest-first ordering, which sorts the vertices in descending order by their degrees.[16]
With the degeneracy ordering, the greedy coloring will use at most d + 1 colors. This is because, when colored, each vertex will have at most d already-colored neighbors, so one of the first d + 1 colors will be free for it to use.[17] Greedy coloring with the degeneracy ordering can find optimal colorings for certain classes of graphs, including trees, pseudoforests, and crown graphs.[18] (Markossian Gasparian) define a graph [math]\displaystyle{ G }[/math] to be [math]\displaystyle{ \beta }[/math]-perfect if, for [math]\displaystyle{ G }[/math] and every induced subgraph of [math]\displaystyle{ G }[/math], the chromatic number equals the degeneracy plus one. For these graphs, the greedy algorithm with the degeneracy ordering is always optimal.[19] Every [math]\displaystyle{ \beta }[/math]-perfect graph must be an even-hole-free graph, because even cycles have chromatic number two and degeneracy two, not matching the equality in the definition of [math]\displaystyle{ \beta }[/math]-perfect graphs. If a graph and its complement graph are both even-hole-free, they are both [math]\displaystyle{ \beta }[/math]-perfect. The graphs that are both perfect graphs and [math]\displaystyle{ \beta }[/math]-perfect graphs are exactly the chordal graphs. On even-hole-free graphs more generally, the degeneracy ordering approximates the optimal coloring to within at most twice the optimal number of colors; that is, its approximation ratio is 2.[20] On unit disk graphs its approximation ratio is 3.[21] The triangular prism is the smallest graph for which one of its degeneracy orderings leads to a non-optimal coloring, and the square antiprism is the smallest graph that cannot be optimally colored using any of its degeneracy orderings.[18]
Adaptive orders
(Brélaz 1979) proposes a strategy, called DSatur, for vertex ordering in greedy coloring that interleaves the construction of the ordering with the coloring process. In his version of the greedy coloring algorithm, the next vertex to color at each step is chosen as the one with the largest number of distinct colors in its neighborhood. In case of ties, a vertex of maximal degree in the subgraph of uncolored vertices is chosen from the tied vertices. By keeping track of the sets of neighboring colors and their cardinalities at each step, it is possible to implement this method in linear time.[22]
This method can find the optimal colorings for bipartite graphs,[23] all cactus graphs, all wheel graphs, all graphs on at most six vertices, and almost every [math]\displaystyle{ k }[/math]-colorable graph.[24] Although (Lévêque Maffray) originally claimed that this method finds optimal colorings for the Meyniel graphs, they later found a counterexample to this claim.[25]
Alternative color selection schemes
It is possible to define variations of the greedy coloring algorithm in which the vertices of the given graph are colored in a given sequence but in which the color chosen for each vertex is not necessarily the first available color. These include methods in which the uncolored part of the graph is unknown to the algorithm, or in which the algorithm is given some freedom to make better coloring choices than the basic greedy algorithm would.
Online selection
Alternative color selection strategies have been studied within the framework of online algorithms. In the online graph-coloring problem, vertices of a graph are presented one at a time in an arbitrary order to a coloring algorithm; the algorithm must choose a color for each vertex, based only on the colors of and adjacencies among already-processed vertices. In this context, one measures the quality of a color selection strategy by its competitive ratio, the ratio between the number of colors it uses and the optimal number of colors for the given graph.[26]
If no additional restrictions on the graph are given, the optimal competitive ratio is only slightly sublinear.[27] However, for interval graphs, a constant competitive ratio is possible,[28] while for bipartite graphs and sparse graphs a logarithmic ratio can be achieved. Indeed, for sparse graphs, the standard greedy coloring strategy of choosing the first available color achieves this competitive ratio, and it is possible to prove a matching lower bound on the competitive ratio of any online coloring algorithm.[26]
Parsimonous coloring
A parsimonious coloring, for a given graph and vertex ordering, has been defined to be a coloring produced by a greedy algorithm that colors the vertices in the given order, and only introduces a new color when all previous colors are adjacent to the given vertex, but can choose which color to use (instead of always choosing the smallest) when it is able to re-use an existing color. The ordered chromatic number is the smallest number of colors that can be obtained for the given ordering in this way, and the ochromatic number is the largest ordered chromatic number among all vertex colorings of a given graph. Despite its different definition, the ochromatic number always equals the Grundy number.[29]
Applications
Because it is fast and in many cases can use few colors, greedy coloring can be used in applications where a good but not optimal graph coloring is needed. One of the early applications of the greedy algorithm was to problems such as course scheduling, in which a collection of tasks must be assigned to a given set of time slots, avoiding incompatible tasks being assigned to the same time slot.[4] It can also be used in compilers for register allocation, by applying it to a graph whose vertices represent values to be assigned to registers and whose edges represent conflicts between two values that cannot be assigned to the same register.[30] In many cases, these interference graphs are chordal graphs, allowing greedy coloring to produce an optimal register assignment.[31]
In combinatorial game theory, for an impartial game given in explicit form as a directed acyclic graph whose vertices represent game positions and whose edges represent valid moves from one position to another, the greedy coloring algorithm (using the reverse of a topological ordering of the graph) calculates the nim-value of each position. These values can be used to determine optimal play in any single game or any disjunctive sum of games.[32]
For a graph of maximum degree Δ, any greedy coloring will use at most Δ + 1 colors. Brooks' theorem states that with two exceptions (cliques and odd cycles) at most Δ colors are needed. One proof of Brooks' theorem involves finding a vertex ordering in which the first two vertices are adjacent to the final vertex but not adjacent to each other, and each vertex other than the last one has at least one later neighbor. For an ordering with this property, the greedy coloring algorithm uses at most Δ colors.[33]
Notes
- ↑ Mitchem (1976).
- ↑ 2.0 2.1 (Hoàng Sritharan), Theorem 28.33, p. 738; (Husfeldt 2015), Algorithm G
- ↑ 3.0 3.1 Frieze & McDiarmid (1997).
- ↑ 4.0 4.1 Welsh & Powell (1967).
- ↑ (Johnson 1974); (Husfeldt 2015).
- ↑ (Kučera 1991); (Husfeldt 2015).
- ↑ Husfeldt (2015).
- ↑ Maffray (2003).
- ↑ Rose, Lueker & Tarjan (1976).
- ↑ (Chvátal 1984); (Husfeldt 2015).
- ↑ Middendorf & Pfeiffer (1990).
- ↑ 12.0 12.1 12.2 12.3 Zaker (2006).
- ↑ Christen & Selkow (1979).
- ↑ (Mitchem 1976); (Husfeldt 2015).
- ↑ Matula & Beck (1983).
- ↑ (Welsh Powell); (Husfeldt 2015).
- ↑ (Matula 1968); (Szekeres Wilf).
- ↑ 18.0 18.1 Kosowski & Manuszewski (2004).
- ↑ (Markossian Gasparian); (Maffray 2003).
- ↑ Markossian, Gasparian & Reed (1996).
- ↑ Gräf, Stumpf & Weißenfels (1998).
- ↑ (Brélaz 1979); (Lévêque Maffray).
- ↑ Brélaz (1979).
- ↑ Janczewski et al. (2001).
- ↑ Lévêque & Maffray (2005).
- ↑ 26.0 26.1 Irani (1994).
- ↑ (Lovász Saks); (Vishwanathan 1992).
- ↑ Kierstead & Trotter (1981).
- ↑ (Simmons 1982); (Erdős Hare).
- ↑ (Poletto Sarkar). Although Poletto and Sarkar describe their register allocation method as not being based on graph coloring, it appears to be the same as greedy coloring.
- ↑ Pereira & Palsberg (2005).
- ↑ E.g., see Section 1.1 of (Nivasch 2006).
- ↑ Lovász (1975).
References
- Brélaz, Daniel (April 1979), "New methods to color the vertices of a graph", Communications of the ACM 22 (4): 251–256, doi:10.1145/359094.359101
- Christen, Claude A.; Selkow, Stanley M. (1979), "Some perfect coloring properties of graphs", Journal of Combinatorial Theory, Series B 27 (1): 49–59, doi:10.1016/0095-8956(79)90067-4
- "Perfectly orderable graphs", Topics in Perfect Graphs, Annals of Discrete Mathematics, 21, Amsterdam: North-Holland, 1984, pp. 63–68. As cited by (Maffray 2003).
- "On the equality of the Grundy and ochromatic numbers of a graph", Journal of Graph Theory 11 (2): 157–159, 1987, doi:10.1002/jgt.3190110205, https://users.renyi.hu/~p_erdos/1987-18.pdf.
- "Algorithmic theory of random graphs", Random Structures & Algorithms 10 (1–2): 5–42, 1997, doi:10.1002/(SICI)1098-2418(199701/03)10:1/2<5::AID-RSA2>3.3.CO;2-6.
- Gräf, A.; Stumpf, M.; Weißenfels, G. (1998), "On coloring unit disk graphs", Algorithmica 20 (3): 277–293, doi:10.1007/PL00009196.
- Hoàng, Chinh T.; Sritharan, R. (2016), "Chapter 28. Perfect Graphs", in Thulasiraman, Krishnaiyan; Arumugam, Subramanian; Brandstädt, Andreas et al., Handbook of Graph Theory, Combinatorial Optimization, and Algorithms, Chapman & Hall/CRC Computer and Information Science Series, 34, CRC Press, pp. 707–750, ISBN 9781420011074.
- Husfeldt, Thore (2015), "Graph colouring algorithms", in Beineke, Lowell W., Topics in Chromatic Graph Theory, Encyclopedia of Mathematics and its Applications, 156, Cambridge University Press, pp. 277–303
- Irani, Sandy (1994), "Coloring inductive graphs on-line", Algorithmica 11 (1): 53–72, doi:10.1007/BF01294263.
- Janczewski, R.; Kubale, M.; Manuszewski, K.; Piwakowski, K. (2001), "The smallest hard-to-color graph for algorithm DSATUR", Discrete Mathematics 236 (1–3): 151–165, doi:10.1016/S0012-365X(00)00439-8.
- Kierstead, H. A. (1981), "An extremal problem in recursive combinatorics", Congressus Numerantium 33: 143–153. As cited by (Irani 1994).
- Kosowski, Adrian; Manuszewski, Krzysztof (2004), "Classical coloring of graphs", in Kubale, Marek, Graph Colorings, Contemporary Mathematics, 352, Providence, Rhode Island: American Mathematical Society, pp. 1–19, doi:10.1090/conm/352/06369
- Kučera, Luděk (1991), "The greedy coloring is a bad probabilistic algorithm", Journal of Algorithms 12 (4): 674–684, doi:10.1016/0196-6774(91)90040-6.
- "Worst case behavior of graph coloring algorithms", Proceedings of the Fifth Southeastern Conference on Combinatorics, Graph Theory and Computing (Florida Atlantic Univ., Boca Raton, Fla., 1974), Congressus Numerantium, X, Winnipeg, Manitoba: Utilitas Math., 1974, pp. 513–527.
- Lévêque, Benjamin; Maffray, Frédéric (October 2005), "Coloring Meyniel graphs in linear time", in Raspaud, André; Delmas, Olivier, 7th International Colloquium on Graph Theory (ICGT '05), 12–16 September 2005, Hyeres, France, Electronic Notes in Discrete Mathematics, 22, Elsevier, pp. 25–28, doi:10.1016/j.endm.2005.06.005, https://hal.archives-ouvertes.fr/file/index/docid/30179/filename/ColMeyniel.pdf. See also Lévêque, Benjamin; Maffray, Frédéric (January 9, 2006), Erratum : MCColor is not optimal on Meyniel graphs.
- "Three short proofs in graph theory", Journal of Combinatorial Theory, Series B 19 (3): 269–271, 1975, doi:10.1016/0095-8956(75)90089-1.
- "An on-line graph coloring algorithm with sublinear performance ratio", Discrete Mathematics 75 (1–3): 319–325, 1989, doi:10.1016/0012-365X(89)90096-4.
- Maffray, Frédéric (2003), "On the coloration of perfect graphs", Recent Advances in Algorithms and Combinatorics, CMS Books in Mathematics, 11, Springer-Verlag, pp. 65–84, doi:10.1007/0-387-22444-0_3, ISBN 0-387-95434-1.
- Markossian, S. E.; Gasparian, G. S. (1996), "β-perfect graphs", Journal of Combinatorial Theory, Series B 67 (1): 1–11, doi:10.1006/jctb.1996.0030.
- "A min-max theorem for graphs with application to graph coloring", SIAM Review 10 (4): 481–482, 1968, doi:10.1137/1010115.
- "Smallest-last ordering and clustering and graph coloring algorithms", Journal of the ACM 30 (3): 417–427, 1983, doi:10.1145/2402.322385.
- Middendorf, Matthias; Pfeiffer, Frank (1990), "On the complexity of recognizing perfectly orderable graphs", Discrete Mathematics 80 (3): 327–333, doi:10.1016/0012-365X(90)90251-C.
- Mitchem, John (1976), "On various algorithms for estimating the chromatic number of a graph", The Computer Journal 19 (2): 182–183, doi:10.1093/comjnl/19.2.182.
- Nivasch, Gabriel (2006), "The Sprague–Grundy function of the game Euclid", Discrete Mathematics 306 (21): 2798–2800, doi:10.1016/j.disc.2006.04.020.
- Pereira, Fernando Magno Quintão; Palsberg, Jens (2005), "Register allocation via coloring of chordal graphs", in Yi, Kwangkeun, Programming Languages and Systems: Third Asian Symposium, APLAS 2005, Tsukuba, Japan, November 2–5, 2005, Proceedings, Lecture Notes in Computer Science, 3780, Springer, pp. 315–329, doi:10.1007/11575467_21
- Poletto, Massimiliano; Sarkar, Vivek (September 1999), "Linear scan register allocation", ACM Transactions on Programming Languages and Systems 21 (5): 895–913, doi:10.1145/330249.330250.
- Rose, D.; Lueker, George (1976), "Algorithmic aspects of vertex elimination on graphs", SIAM Journal on Computing 5 (2): 266–283, doi:10.1137/0205021.
- Simmons, Gustavus J. (1982), "The ordered chromatic number of planar maps", Congressus Numerantium 36: 59–67
- Sysło, Maciej M. (1989), "Sequential coloring versus Welsh–Powell bound", Discrete Mathematics 74 (1–2): 241–243, doi:10.1016/0012-365X(89)90212-4.
- "An inequality for the chromatic number of a graph", Journal of Combinatorial Theory 4: 1–3, 1968, doi:10.1016/S0021-9800(68)80081-X.
- Vishwanathan, Sundar (1992), "Randomized online graph coloring", Journal of Algorithms 13 (4): 657–669, doi:10.1016/0196-6774(92)90061-G.
- "An upper bound for the chromatic number of a graph and its application to timetabling problems", The Computer Journal 10 (1): 85–86, 1967, doi:10.1093/comjnl/10.1.85.
- Zaker, Manouchehr (2006), "Results on the Grundy chromatic number of graphs", Discrete Mathematics 306 (2–3): 3166–3173, doi:10.1016/j.disc.2005.06.044.
![]() | Original source: https://en.wikipedia.org/wiki/Greedy coloring.
Read more |